Purpose of this Document
This document is designed as part of a series of demo script documents to
assist customers who are evaluating Microsoft® Visual FoxPro™ 5.0. It can
be used by an individual developer learning a new feature in Visual FoxPro
5.0; or as the basis for a demo shown to other developers and software tools
evaluators. This demo script requires Visual FoxPro 5.0 to be installed.
Questions about this demo script should be directed via email to
foxmktg@microsoft.com.
Introduction
ActiveX™ controls (formerly known as OLE controls) are reusable software
components that can be added to existing applications with minimal additional
coding. Using ActiveX controls, developers can add unique functionality to
their applications using prebuilt and pretested components. With over 2,000
ActiveX controls currently available, Visual FoxPro developers can access a
rich inventory of components to extend and enhance their applications (this capability is demonstrated in the documents entitled "Demo Script: Using the ActiveMovie Control in Visual FoxPro 5.0" and "Demo Script: Using the ProgressBar Control in Visual FoxPro 5.0" available from the same location as this document).
Developers can use the tools in Microsoft Visual Studio 97™ or Visual
Basic® Control Creation Edition to create their own redistributable
ActiveX controls.
Visual FoxPro 5.0 further extends the power of ActiveX controls by enabling
the use of ActiveX controls in Visual FoxPro classes. A control's methods and
properties may be manipulated, adding binding to specific data, for example,
and then saved and reused in a Visual FoxPro class library. This approach
speeds development and simplifies application maintenance.
This paper shows you how to demonstrate subclassing an ActiveX control in
Visual FoxPro 5.0. You will take the Calendar control, which is not inherently
data bound and, through code, bind it to a date column in a table.
Show that the Calendar Control is not Data Bound
Create a new form by typing create form in the Command window. In the
form's Data Environment add the Orders table. You can use
\Vfp\Samples\Data\Orders.dbf.
The Visual FoxPro Data Environment is a central design surface used with Forms
and Reports where all major elements of data access can be manipulated. This
includes all of the tables, indexes, relationships, defaults, rules, triggers,
etc., associated with the form or report. In addition, the developer can drag
and drop fields and even entire tables from the Data Environment window to the
form or report, speeding and simplifying form creation.
Drag a few of the fields from the Orders table onto the form. Include the
field order_date.
In the Form Controls toolbar click on the View Classes button and then
choose Add from the popup menu. In the Open dialog locate the
file \Vfp\Samples\Classes\Buttons.vcx and select it. The toolbar will change
to show the classes in this class library, which contains various buttons for
use on forms. Add the vcr class to the form. This provides generic
navigation buttons and will allow you to scroll from record to record.
Click on the View Classes button and
choose Standard from the popup menu. This will set the toolbar back to
its default.
Click on the OLE Container Control button.
Position the mouse cursor where you want the control to be and then drag until
you have a rectangle. In the Insert Object dialog choose Insert
Control. The Control Type list then shows all of the ActiveX controls
registered on your machine. Choose Calendar Control from the list and
then choose OK. Resize the control on the form so you can see the whole
calendar.
In the Properties window notice that there are properties for Day, Month,
and Year but there is no ControlSource property for the Calendar
control. You would like to able to bind the Calendar control directly to the order_date
column, but you can't.
Run the form and save it as Calendar.scx. As you scroll from record to record
you will see that the calendar displays today's date, and never changes. Close
the form.
Create the Calendar Control Subclass
Create a new class by typing create class in the Command window. In the
New Class dialog enter databoundcalendar as the name of the
class. Choose OLEControl from the Based On list. Then store the new
class in the file Activex.vcx. Choose OK.
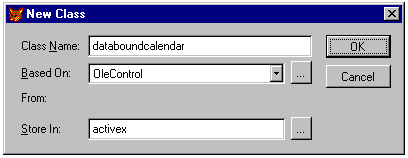
By encapsulating the control in a Visual FoxPro class, you are creating a
reusable object that inherits the full power of the Visual FoxPro object
oriented architecture. That is, you may reuse the object in many places, even
alter its behavior with each use, and still maintain a link back to the base
object. This makes global changes and maintenance of your objects much simpler
than ever before. (For more information on the object oriented features in
Visual FoxPro 5.0, please see the white paper entitled, "Creating Reusable
Classes with Visual FoxPro," found on the Visual FoxPro Web site.)
When the New Class dialog is closed, the Class Designer is opened and the Insert Object dialog appears. In the Insert Object dialog choose Insert Control. Choose Calendar
Control from the Control Type list and then choose OK. Resize the
control in the Class Designer so you can see the whole calendar.
Choose New Property from the Class menu and add a property
called date_column to the class. Choose New Method from the Class
menu and add a method called RefreshDisplay.
Add custom code to the Calendar control to make it data bound
Add the following code to the RefreshDisplay method. This code will set
the Month, Day, and Year properties of the Calendar
control based on the value in the date field used to bind the control.
* What column is the control bound to?
cColumn = This.date_column
* If it is bound to a column
IF NOT EMPTY(cColumn)
* Set the Month, Day, and Year properties of the
* calendar control to the Month, Day, and Year
* of the date column it is bound to
This.Month = Month(&cColumn)
This.Day = Day(&cColumn)
This.Year = Year(&cColumn)
ENDIF
Add the following code to the AfterUpdate method. This method is called
automatically when you change the date in the calendar. It constructs a Visual
FoxPro date variable from the appropriate properties of the Calendar control
and then stores that value in the date field used to bind the control.
* What column is the control bound to?
cColumn = This.date_column
* Construct a Visual FoxPro style date from the Month,
* Day, and Year properties of the Calendar
dValue = CTOD(ALLTRIM(STR(This.Month)) + "/" + ;
ALLTRIM(STR(This.Day)) + "/" + ;
ALLTRIM(STR(This.Year)))
* If the Calendar is bound to a column
IF NOT EMPTY(cColumn)
* Update the value of the date field with the date
* derived from the Calendar control
Replace &cColumn With dValue
ENDIF
ThisForm.Refresh
Close and save the class.
Use the Subclassed Control on a Form
Open the form and delete the Calendar control. Click on the View Classes
button on the Form Controls toolbar and choose Add from the popup menu.
In the Open dialog locate the file Activex.vcx and select it. The
toolbar will change to show the icon for the databoundcalendar class
you just created. Add the class to the form and size it so you can see the
entire calendar.
Name the Calendar control ocxCalendar. Set the date_column
property of the control to orders.order_date. (Hint: The Other tab in
the Properties window displays both the Name and date_column properties.)
Add the following code to the Refresh method of the form. This will
cause the control's RefreshDisplay method to run whenever the form is
refreshed.
* Run the refresh method of the Calendar control,
* which will update the date in the Calendar
ThisForm.ocxCalendar.RefreshDisplay
Save and run the form. The calendar is now bound to the order_date
field in the Orders table. As you scroll from record to record the date
on the calendar changes. Also, if you change the date in the calendar that
change is reflected in the data.
Behind the Scenes
The Calendar control is not inherently data bound, even though it has
properties for month, day, and year. When you select a date on the calendar
you are setting these properties of the control. To make the control data
bound you merely need to write some code to map a date field in a Visual
FoxPro table to the month, day, and year properties of the control. Writing
this code in a class means that you don't have to write it every time you want
to use the Calendar control on a form.
As you scroll from record to record you call the RefreshDisplay method
(via the form's Refresh method) to ensure the Calendar control displays
the proper date. The code you put in that method of the databoundcalendar
class reads a date field and sets the month, day, and year properties of the
Calendar control accordingly.
The AfterUpdate method is called if you use the Calendar to change the
date. The code you put in that method updates the date field based on the
values of the control's properties.
Summary
ActiveX controls are a powerful way of reusing components to speed application
development and simplify maintenance. Support in Visual FoxPro 5.0 for object
oriented programming enables visual subclassing of ActiveX controls. This
further extends the power of ActiveX, and gives the Visual FoxPro developer
very fine-grained control over the development process.
Frequently-Asked Questions
How can I learn more about using ActiveX controls with Visual FoxPro 5.0?
A good place to start is the white paper entitled, "ActiveX Controls and
Visual FoxPro 5.0," found on the Visual FoxPro Web site. Also, see Chapter 16,
"Adding OLE," in the Developer's Guide, part of the documentation
that came with your copy of Visual FoxPro 5.0.
Can Visual FoxPro 5.0 create ActiveX controls?
Visual FoxPro is an application development environment that focuses on
assembling applications from components, rather than on building components
per se. Microsoft provides several tools for component creation (Visual
C++®, Visual Basic®, Visual Basic - Control Creation Edition, Visual
J++™).
What other products can use ActiveX controls?
All of the tools in Visual Studio 97™, plus Microsoft® Office 97 and
Microsoft® Internet Explorer. ActiveX controls are a standard component of
Windows, so many other third-party products support them as well.
How are a control's properties and methods documented?
In many cases, controls install with help files (.HLP) which describe how to
program them. In cases where no help files are included, developers can use an
Object Browser which ships with several other Microsoft tools, to inspect the
control's properties, events, and methods.
Can I obtain an evaluation copy of Visual FoxPro 5.0?
Visual FoxPro 5.0, and all Microsoft products, are available with a 30-day
money back guarantee from software resellers. Therefore, if you purchase the
product on a trial basis, and decide not to keep it, simply return it for a
full refund.
How do I get the Visual Basic Control Creation Edition (VBCCE)?
Visual Basic - Control Creation Edition is free, available for download.
Please see www.microsoft.com/vbasic/ for more information.